Latest Update needs https to work (dont verify the cert or safe it via a web browser). Also the Display does no longer work and the STATISTIC variables are all gone.
Hint: To get STATISTIC values you can use the API for meinsenec.de, https://documenter.getpostman.com/view/10329335/UVCB9ihW#17e2c6c6-fe5e-4ca9-bc2f-dca997adaf90
For a personal project I wanted to show the current status of a photovoltaic system made by Senec on a small display in the living room. Till then I used an old Android smartphone with a disabled screensaver, which showed the local webinterface.
To get to the webinterface in a browser you can use the following link, which will give you a website like shwon below.
https://SENEC_IP/
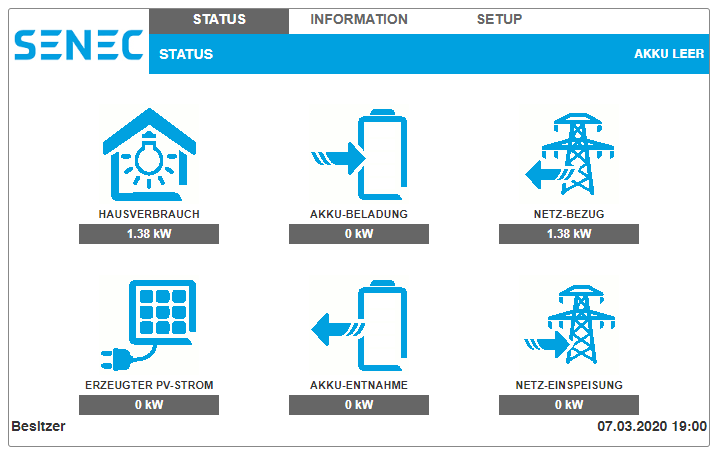
Another interesting link show you a representation of the display. So you don't have to check the appliance yourself.
https://SENEC_IP/Display.html
Edit: this is broken as of September 2023, the Display will stay empty
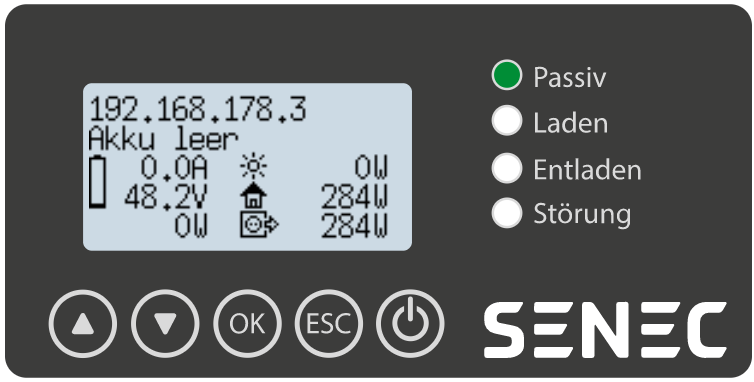
Finding the hidden API
To get this working I needed to know how the local webinterface gets its data. This was much easier than expected! Using the Developer Tools in Chrome, expecially the Network tab, I was able to see a POST-request which was made repeatedly.
Then I tried to mimic this request with Postman, which worked instantly for some parameters. After some testing I noticed, the content of the POST-Request is important! It determines the size of the response. To get all possible sections for the request you can POST the following.
Request Type:
POST https://YOUR_SENEC_IP/lala.cgi
Header:
No special headers required
Body:
{
"DEBUG": {}
}
As a response you will get a list of all the possible sections you can include in your request. See SECTIONS below:
{
"DEBUG": {
"CHARGE_TARGET": "fl_00000000",
"DC_TARGET": "fl_00000000",
"DC_TARGET_PID_KD": "fl_3CA3D70A",
"DC_TARGET_PID_KI": "fl_3C23D70A",
"DC_TARGET_PID_KP": "fl_3E4CCCCD",
"EG_POT_BOOST_CAP": "fl_00000000",
"EG_POT_BOOST_POW": "fl_00000000",
"EG_POT_CHARGE_CAP": "fl_4590F933",
"EG_POT_CHARGE_POW": "fl_44907195",
"FEED_TARGET": "fl_43AF5C05",
"PU_AVAIL": "fl_00000000",
"SECTIONS": [
"st_BAT1",
"st_BAT1OBJ1",
"st_BMS",
"st_CASC",
"st_CONNX50",
"st_DEBUG",
"st_DISPLAY",
"st_EG_CONTROL",
"st_ENERGY",
"st_FACTORY",
"st_FEATURES",
"st_FILE",
"st_GRIDCONFIG",
"st_ISKRA",
"st_LOG",
"st_PM1",
"st_PM1OBJ1",
"st_PM1OBJ2",
"st_PV1",
"st_PWR_UNIT",
"st_RTC",
"st_SOCKETS",
"st_STATISTIC",
"st_STECA",
"st_SYS_UPDATE",
"st_TEMPMEASURE",
"st_TEST",
"st_UPDATE",
"st_WALLBOX",
"st_WIZARD"
]
}
}
The following is a sample request to get a lot of different data points. You mix and match the sections you want/need for your project. But remember, that the response will take more time the more sections you request.
Request Type:
POST https://YOUR_SENEC_IP/lala.cgi
Header:
No special headers required
Body:
{
"ENERGY": {},
"PV1": {},
"PM1OBJ1":{},
"STATISTIC": {},
"RTC": {},
"LICENSE": {},
"LOG": {},
"WIZARD": {},
"BMS": {},
"FACTORY": {},
"DISPLAY": {}
}
You could also shorten the list of parameters you want to query. This will speed up the process a lot! e.g.:
Request Type:
POST http://YOUR_SENEC_IP/lala.cgi
Header:
No special headers required
Body:
{
"ENERGY": {
"GUI_BAT_DATA_POWER": "",
"GUI_BAT_DATA_FUEL_CHARGE": "",
"GUI_INVERTER_POWER": "",
"GUI_HOUSE_POW": "",
"GUI_GRID_POW": "",
"OFFPEAK_TARGET": "",
"STAT_HOURS_OF_OPERATION": ""
}
}
The answer to this will look somethin like this:
{
"ENERGY": {
"GUI_BAT_DATA_POWER": "fl_C3BAD251",
"GUI_BAT_DATA_FUEL_CHARGE": "fl_42A2E5E4",
"GUI_INVERTER_POWER": "fl_80000000",
"GUI_HOUSE_POW": "fl_43B24272",
"GUI_GRID_POW": "fl_C04851EB",
"OFFPEAK_TARGET": "u8_64",
"STAT_HOURS_OF_OPERATION": "u3_0000183E"
}
}
Next I will show you how to interpret these values und convert them on a Arduino based board!
Interpreting the parameters
The format of these values is always the same. It starts with two characters which represent the type of data transferred. For example fl_
means float
and u8_
means uint8
which is a 8bit unsigned (one byte) integer array.
This definition is followed by the value represented in Hexadecimal, not Decimal. So you just have to convert hex
to dec
. An example:
"GUI_BAT_DATA_FUEL_CHARGE": "fl_42A2E5E4"
fl_
means this is a float value composed of 4 bytes.42A2E5E4
is the value written in hexadecimal, representing the 4 bytes of a float.
If you use an online converter like https://gregstoll.com/~gregstoll/floattohex/ you will get the following value: 81.449
. This means the battery of the Senec photovoltaic appliance is currently charged to 81.449%.
Another example would be "OFFPEAK_TARGET": "u8_64"
which, following the above logic, results in a unsignd int of 8 bits or a byte. 64
in hex represents 100
in decimal.
The last one would be "STAT_HOURS_OF_OPERATION": "u3_0000183E"
which is a 32bit/4 byte unsigned integer. After conversion from hex to int this value reads 6206 hours of operation.
Custom functions for an ESP32/Arduino
To convert a fl_
value I am using the following custom function. This function uses a union to cast the bit values of a byte array into a float value and atoh()
to create decimal values from the hex strings. (see comments inline)
float convertFloat(const char *text){
//create a union to easily cast values into another type
union floatData{
byte by[4];
float fl;
} dataValue;
//get number of bytes (4 for float values)
byte bytes = (strlen(text)-3)/2;
//filling the byte array
for(int i=0;i<bytes;i++){
dataValue.by[3-i]=atoh(text[2*i+3]);
dataValue.by[3-i]=dataValue.by[3-i]<<4;
dataValue.by[3-i]=dataValue.by[3-i]+atoh(text[2*i+4]);
}
//returning the byte array as a float through the union.
return dataValue.fl;
}
//hex string to decimal
unsigned char atoh (unsigned char data){
if (data > '9')
{ data += 9;
}
return (data &= 0x0F);
}
The same for 8bit uint8
/ u8_
:
byte convertByte(const char *text){
union byteData{
byte by;
} dataValue;
byte bytes = (strlen(text)-3)/2;
for(int i=0;i<bytes;i++){
dataValue.by=atoh(text[2*i+3]);
dataValue.by=dataValue.by<<4;
dataValue.by=dataValue.by+atoh(text[2*i+4]);
}
return dataValue.by;
}
And also for 16bit int
/ u1_
:
int convertInt(const char *text){
union intData{
byte by[2];
float in;
} dataValue;
byte bytes = (strlen(text)-3)/2;
for(int i=0;i<bytes;i++){
dataValue.by[1-i]=atoh(text[2*i+3]);
dataValue.by[1-i]=dataValue.by[i]<<4;
dataValue.by[1-i]=dataValue.by[i]+atoh(text[2*i+4]);
}
return dataValue.in;
}
And also for 16bit int
/ u3_
:
unsigned long convertUnsignedLong(const char *text){
union inData{
byte input[4];
unsigned long output;
} dataValue;
byte bytes = (strlen(text)-3)/2;
for(int i=0;i<bytes;i++){
dataValue.input[3-i]=atoh(text[2*i+3]);
dataValue.input[3-i]=dataValue.input[3-i]<<4;
dataValue.input[3-i]=dataValue.input[3-i]+atoh(text[2*i+4]);
}
return dataValue.output;
}
Now you should have gotten the hang of it. The other values can be translated in the same manner. Just put the Hex values into a byte array and then cast it to the wanted datatype using a union. Maybe one could even create a funktion which decides the correct datatype on its own.
In my next post I can hopefully present you my full implementation of a Senec status display using a LED matrix and an ESP32.
Bonus: long example response with all parameters:
{
"ENERGY": {
"CAPTESTMODULE": [
"fl_00000000",
"fl_00000000",
"fl_00000000",
"fl_00000000"
],
"GRID_POWER_OFFSET": "fl_00000000",
"GUI_BAT_DATA_COLLECTED": "u8_01",
"GUI_BAT_DATA_CURRENT": "fl_C0D00000",
"GUI_BAT_DATA_FUEL_CHARGE": "fl_42A705A2",
"GUI_BAT_DATA_POWER": "fl_C3B4D979",
"GUI_BAT_DATA_VOLTAGE": "fl_425E9581",
"GUI_BOOSTING_INFO": "u8_01",
"GUI_CAP_TEST_DIS_COUNT": "fl_00000000",
"GUI_CAP_TEST_START": "u8_00",
"GUI_CAP_TEST_STATE": "u8_00",
"GUI_CAP_TEST_STOP": "u8_00",
"GUI_CHARGING_INFO": "u8_00",
"GUI_FACTORY_TEST_FAN": "u8_00",
"GUI_FACTORY_TEST_RELAY": "u8_00",
"GUI_GRID_POW": "fl_C11570A4",
"GUI_HOUSE_POW": "fl_43A42F9E",
"GUI_INIT_CHARGE_START": "u8_00",
"GUI_INIT_CHARGE_STOP": "u8_00",
"GUI_INVERTER_POWER": "fl_80000000",
"GUI_TEST_CHARGE_STAT": "u8_00",
"GUI_TEST_DISCHARGE_STAT": "u8_00",
"INIT_CHARGE_ACK": "u8_00",
"INIT_CHARGE_DIFF_VOLTAGE": "fl_00000000",
"INIT_CHARGE_MAX_CURRENT": "fl_00000000",
"INIT_CHARGE_MAX_VOLTAGE": "fl_00000000",
"INIT_CHARGE_MIN_VOLTAGE": "fl_00000000",
"INIT_CHARGE_RERUN": "u8_00",
"INIT_CHARGE_RUNNING": "u8_00",
"INIT_CHARGE_STATE": "u8_00",
"INIT_CHARGE_TIMER": "u6_0000000000000000",
"INIT_DISCHARGE_MAX_CURRENT": "fl_00000000",
"LI_STORAGE_MODE_RUNNING": "u8_00",
"LI_STORAGE_MODE_START": "u8_00",
"LI_STORAGE_MODE_STOP": "u8_00",
"OFFPEAK_DURATION": "u1_0000",
"OFFPEAK_POWER": "fl_00000000",
"OFFPEAK_RUNNING": "u8_00",
"OFFPEAK_TARGET": "u8_64",
"SAFE_CHARGE_FORCE": "u8_00",
"SAFE_CHARGE_PROHIBIT": "u8_00",
"SAFE_CHARGE_RUNNING": "u8_00",
"STAT_HOURS_OF_OPERATION": "u3_0000183E",
"STAT_LIMITED_NET_SKEW": "u8_00",
"STAT_STATE": "u8_10",
"STAT_STATE_DECODE": "u8_10",
"TEST_CHARGE_CHRG_START": "u8_00",
"TEST_CHARGE_CHRG_STOP": "u8_01",
"TEST_CHARGE_DIS_START": "u8_00",
"TEST_CHARGE_DIS_STOP": "u8_01",
"TEST_CYCLE": "u8_00",
"ZERO_EXPORT": "u8_00"
},
"PV1": {
"ERROR_STATE_INT": [
"u3_00000000",
"u3_00000000"
],
"INTERNAL_INV_ERROR_TEXT": [
"st_",
"st_"
],
"INTERNAL_INV_ERR_STATE_VALID": [
"u8_01",
"u8_00"
],
"INTERNAL_INV_STATE": [
"u8_02",
"u8_05"
],
"INTERNAL_MD_AVAIL": [
"u8_01",
"u8_00"
],
"INTERNAL_MD_MANUFACTURER": [
"st_Senec",
"st_"
],
"INTERNAL_MD_MODEL": [
"st_V3 LV",
"st_"
],
"INTERNAL_MD_SERIAL": [
"st_XXXXXXXXXXXXXXXX",
"st_"
],
"INTERNAL_MD_VERSION": [
"st_HMI: X.XX.XX PU: X.X.XX BDC: X.XX.X",
"st_"
],
"INTERNAL_PV_AVAIL": "u8_01",
"INV_MODEL": [
"st_",
"st_END_OF_ARRAY"
],
"INV_SERIAL": [
"st_",
"st_END_OF_ARRAY"
],
"INV_VERSIONS": [
"st_HMI: X.XX.XX PU: X.X.XX",
"st_END_OF_ARRAY"
],
"MPP_AVAIL": "u8_02",
"MPP_CUR": [
"fl_BE408313",
"fl_BE116873",
"fl_00000000"
],
"MPP_POWER": [
"fl_C158F9DC",
"fl_C126D0E6",
"fl_00000000"
],
"MPP_VOL": [
"fl_42982561",
"fl_429B6354",
"fl_00000000"
],
"POWER_RATIO": "u3_00000064",
"PV_MISSING": "u8_01",
"P_TOTAL": "fl_41BFE561",
"STATE_INT": [
"u3_00000110",
"u3_00000000"
],
"TYPE": "u8_A0"
},
"PM1OBJ1": {
"ADR": "u8_01",
"ENABLED": "u8_01",
"FREQ": "fl_42483D70",
"I_AC": [
"fl_3F866666",
"fl_3EA8F5C2",
"fl_3F3851EB"
],
"P_AC": [
"fl_C32D0000",
"fl_3F7D70A3",
"fl_4322547B"
],
"P_TOTAL": "fl_C11AB852",
"U_AC": [
"fl_43666667",
"fl_43680000",
"fl_43676667"
]
},
"STATISTIC": {
"CURRENT_STATE": "u1_0010",
"LIVE_BAT_CHARGE": "fl_44313EF4",
"LIVE_BAT_CHARGE_MASTER": "fl_44313EF4",
"LIVE_BAT_DISCHARGE": "fl_442AA859",
"LIVE_BAT_DISCHARGE_MASTER": "fl_442AA859",
"LIVE_GRID_EXPORT": "fl_4501C7BE",
"LIVE_GRID_IMPORT": "fl_438C942B",
"LIVE_HOUSE_CONS": "fl_44BF0FE3",
"LIVE_PU_ENERGY": [
"fl_00000000",
"fl_00000000",
"fl_00000000",
"fl_00000000",
"fl_00000000",
"fl_00000000"
],
"LIVE_PV_GEN": "fl_45526CA0",
"LIVE_WB_ENERGY": [
"u6_0000000000000000",
"u6_0000000000000000",
"u6_0000000000000000",
"u6_0000000000000000"
],
"MEASURE_TIME": "i3_5FE0C82D",
"STAT_DATA_PUBLISHED": "VARIABLE_NOT_FOUND",
"STAT_REQ_CLEAR_REM_DATA": "u8_00",
"STAT_SUM_E_PU": [
"u6_0000000000000000",
"u6_0000000000000000",
"u6_0000000000000000",
"u6_0000000000000000",
"u6_0000000000000000",
"u6_0000000000000000"
]
},
"RTC": {
"TIMESTAMP_MS": "u3_1995C856",
"UTC_OFFSET": "i3_0000003C",
"WEB_TIME": "u3_5FE0D63D"
},
"LOG": {
"LOG_IN_BUTT": "u8_00",
"LOG_OUT_BUTT": "u8_00",
"PASSWORD": "st_",
"USERNAME": "st_",
"USER_LEVEL": "u8_00"
},
"WIZARD": {
"APPLICATION_HASH": "st_XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"APPLICATION_VERSION": "st_XXXX",
"BOOT": "u8_01",
"CHARGE_PRIO": "u8_00",
"CONFIG_CHECKSUM": "u1_XXXX",
"CONFIG_LOADED": "u8_01",
"CONFIG_MODIFIED_BY_USER": "u8_00",
"CONFIG_WRITE": "u8_00",
"DEVICE_BATTERY_TYPE": "u8_04",
"DEVICE_INVERTER_TYPE": "u8_A0",
"DEVICE_INV_ENABLED": [
"u8_01",
"u8_00",
"u8_00",
"u8_00",
"u8_00",
"u8_00"
],
"DEVICE_INV_PHASES_ARR": [
"u8_04",
"u8_04",
"u8_04",
"u8_04",
"u8_04",
"u8_04"
],
"DEVICE_INV_SLAVE_ADRESS": [
"u8_03",
"u8_04",
"u8_05",
"u8_06",
"u8_07",
"u8_08"
],
"DEVICE_PM_GRID_ENABLED": "u8_01",
"DEVICE_PM_HOUSE_ENABLED": "u8_00",
"DEVICE_PM_TYPE": "u8_01",
"FEATURECODE_ENTERED": "u8_00",
"FIRMWARE_VERSION": "st_XXX",
"GENERATION_METER_SN": "u3_00000000",
"GRID_CONNECTION_TYPE": "u8_02",
"GUI_LANG": "u8_00",
"HEATPUMP_METER_SN": "u3_XXXXXXXX",
"HEAT_CONN_TYPE": "u8_02",
"INSULATION_RESISTANCE": "i3_000003E8",
"INTERFACE_VERSION": "st_XXXX",
"MAC_ADDRESS_BYTES": [
"u8_XX",
"u8_XX",
"u8_XX",
"u8_XX",
"u8_XX",
"u8_XX"
],
"MASTER_SLAVE_ADDRESSES": [
"u8_00",
"u8_00",
"u8_00",
"u8_00",
"u8_00",
"u8_00"
],
"MASTER_SLAVE_MODE": "u8_00",
"NET_DNS_SERVER_IP": "u3_XXXXXXX",
"NET_GATEWAY_IP": "u3_XXXXXXXX",
"NET_NETWORK_MASK": "u3_XXXXXXXX",
"NET_SENEC_NO_NETWORK": "u8_00",
"NET_SENEC_SERVER_IP": "u3_XXXXXXXX",
"NET_SENEC_STATIC_IP": "u3_XXXXXXXX",
"NET_SENEC_USE_DHCP": "u8_00",
"PS_ENABLE": "u8_00",
"PS_HOUR": "u8_00",
"PS_MINUTE": "u8_00",
"PS_RESERVOIR": "u8_00",
"PV_CONFIG": [
"u8_01",
"u8_01"
],
"PWRCFG_PEAK_PV_POWER": "fl_45796000",
"SENEC_METER_SN": "u3_XXXXXXXX",
"SETUP_ABS_POWER": "u3_00000000",
"SETUP_AGBS_ACCEPTED": "u8_00",
"SETUP_HV_PHASE": "u8_00",
"SETUP_NUMBER_WALLBOXES": "u8_00",
"SETUP_PM_GRID_ADR": "u8_01",
"SETUP_PM_HOUSE_ADR": "u8_02",
"SETUP_POWER_RULE": "u8_46",
"SETUP_PV_INV_IP0": "u3_00000000",
"SETUP_PV_INV_IP1": "u3_00000000",
"SETUP_PV_INV_IP2": "u3_00000000",
"SETUP_PV_INV_IP3": "u3_00000000",
"SETUP_PV_INV_IP4": "u3_00000000",
"SETUP_PV_INV_IP5": "u3_00000000",
"SETUP_RCR_STEPS": [
"u1_0000",
"u1_001E",
"u1_003C",
"u1_0064"
],
"SETUP_USED_PHASE": "u8_01",
"SETUP_USE_ABS_POWER": "u8_00",
"SETUP_USE_RCR": "u8_00",
"SETUP_WALLBOX_IDS": [
"u8_01",
"u8_02",
"u8_03",
"u8_04"
],
"SETUP_WALLBOX_MAX_TOTAL_CURRENT_BY_GRID": "fl_00000000",
"SETUP_WALLBOX_SERIAL0": "st_",
"SETUP_WALLBOX_SERIAL1": "st_",
"SETUP_WALLBOX_SERIAL2": "st_",
"SETUP_WALLBOX_SERIAL3": "st_",
"SG_READY_CURR_MODE": "u8_01",
"SG_READY_ENABLED": "u8_00",
"SG_READY_EN_MODE1": "u8_00",
"SG_READY_POWER_COMM": "u1_FFFF",
"SG_READY_POWER_PROP": "u1_FFFF",
"SG_READY_TIME": "u1_02D0",
"TEST_GENERATION_METER": "u8_00",
"TEST_HEATPUMP_METER": "u8_00",
"TEST_SENEC_METER": "u8_00",
"USE_TESTSERVER": "u8_00"
},
"BMS": {
"ALARM_STATUS": [
"u1_0000",
"u1_0000",
"u1_0000",
"u1_0000"
],
"BATTERY_STATUS": [
"u1_0000",
"u1_0000",
"u1_0000",
"u1_0000"
],
"BL": [
"u1_2774",
"u1_2774",
"u1_0000",
"u1_0000"
],
"BMS_READY_FLAG": "u8_01",
"CHARGED_ENERGY": [
"u3_15570926",
"u3_155DFE42",
"u3_00000000",
"u3_00000000"
],
"CHARGE_CURRENT_LIMIT": [
"fl_41400000",
"fl_41400000",
"fl_00000000",
"fl_00000000"
],
"COMMERRCOUNT": "u1_0000",
"CURRENT": [
"fl_C05147AE",
"fl_C05147AE",
"fl_00000000",
"fl_00000000"
],
"CYCLES": [
"u1_007A",
"u1_007B",
"u1_0000",
"u1_0000"
],
"DERATING": "u8_00",
"DISCHARGED_ENERGY": [
"u3_148655C2",
"u3_14BCD346",
"u3_00000000",
"u3_00000000"
],
"DISCHARGE_CURRENT_LIMIT": [
"fl_C1BF3333",
"fl_C1BF3333",
"fl_00000000",
"fl_00000000"
],
"ERROR": "u8_00",
"FAULTLINECOUNT": "u1_0000",
"FW": [
"u1_XXXX",
"u1_XXXX",
"u1_0000",
"u1_0000"
],
"HW_EXTENSION": [
"u1_XXXX",
"u1_XXXX",
"u1_0000",
"u1_0000"
],
"HW_MAINBOARD": [
"u1_00XX",
"u1_00XX",
"u1_0000",
"u1_0000"
],
"MANUFACTURER": "u8_02",
"MAX_CELL_VOLTAGE": [
"u1_0F95",
"u1_0F93",
"u1_0000",
"u1_0000"
],
"MAX_TEMP": "i1_010E",
"MIN_CELL_VOLTAGE": [
"u1_0F74",
"u1_0F72",
"u1_0000",
"u1_0000"
],
"MIN_TEMP": "i1_00E6",
"MODULES_CONFIGURED": "u8_02",
"MODULE_COUNT": "u8_02",
"NOM_CHARGEPOWER_MODULE": "fl_441C4000",
"NOM_DISCHARGEPOWER_MODULE": "fl_449C4000",
"NR_INSTALLED": "u8_02",
"PROTOCOL": "u1_0000",
"RECOVERLOCKED": "u8_00",
"SERIAL": [
"st_",
"st_",
"st_",
"st_"
],
"SN": [
"u3_0000XXXX",
"u3_0000XXXX",
"u3_00000000",
"u3_00000000"
],
"SOC": [
"u1_0055",
"u1_0054",
"u1_0000",
"u1_0000"
],
"SOH": [
"u8_62",
"u8_62",
"u8_00",
"u8_00"
],
"START_SELFTEST": "u8_01",
"START_UPDATE": "u8_00",
"STATUS": [
"u3_00000001",
"u3_00000001",
"u3_00000000",
"u3_00000000"
],
"SYSTEM_SOC": "u1_0348",
"TEMP_MAX": [
"i8_1B",
"i8_1B",
"i8_00",
"i8_00"
],
"TEMP_MIN": [
"i8_17",
"i8_17",
"i8_00",
"i8_00"
],
"VOLTAGE": [
"fl_425EA3D7",
"fl_425E872B",
"fl_00000000",
"fl_00000000"
],
"WIZARD_ABORT": "u8_01",
"WIZARD_CONFIRM": "u8_00",
"WIZARD_DCCONNECT": "u1_0000",
"WIZARD_START": "u8_00",
"WIZARD_STATE": "u8_00"
},
"FACTORY": {
"AUX_TYPE": "u8_03",
"BAT_TYPE": "u8_04",
"BEH_FLAGS": "u8_01",
"CELL_TYPE": "u8_05",
"COUNTRY": "u8_00",
"DESIGN_CAPACITY": "fl_459C4000",
"DEVICE_ID": "st_XXXXXXXXXXXXXXXXXXXXX",
"FAC_SANITY": "u8_01",
"MAX_CHARGE_POWER_DC": "fl_449C4000",
"MAX_DISCHARGE_POWER_DC": "fl_451C4000",
"PM_TYPE": "u8_01",
"SYS_TYPE": "u8_11",
"TEMP_TYPE": "u8_00"
}
}